Python | Java |
---|
01
02
03
04
05
06
07
08
|
def main():
x = "Bob"
print("Hello " + x)
x = 42
print(x - 1)
if __name__ == "__main__":
main()
|
|
01
02
03
04
05
06
07
08
09
10
11
|
package algs11;
import stdlib.*;
public class Hello {
public static void main (String[] args) {
String x;
x = "Bob";
StdOut.println ("Hello " + x);
x = 42; // Compiler error
StdOut.println (x - 1); // Compiler error
}
}
|
|
Python allows a single variable to be used at multiple types.
By typing variables, java catches more errors before runtime.
Python | Java |
---|
01
02
03
04
05
06
|
def main():
x = "Bob"
print(x - 1)
if __name__ == "__main__":
main()
|
|
01
02
03
04
05
06
07
08
09
|
package algs11;
import stdlib.*;
public class Hello {
public static void main (String[] args) {
String x;
x = "Bob";
StdOut.println (x - 1);
}
}
|
|
Java does more implicit conversions than python.
Python | Java |
---|
01
02
03
04
05
06
|
def main():
x = "Bob"
print(x + 1)
if __name__ == "__main__":
main()
|
|
01
02
03
04
05
06
07
08
09
|
package algs11;
import stdlib.*;
public class Hello {
public static void main (String[] args) {
String x;
x = "Bob";
StdOut.println (x + 1);
}
}
|
|
You can safely ignore the rest of this slide, which uses some more
advanced java features that you don't need just yet.
The special class java.lang.Integer
is used to create
objects holding a single integer (more later).
All java objects can be referenced at type Object
.
Using these properties it is possible to mimic the original python
code above, but java requires explicit casts in order to apply the
concatenation and subtraction operators.
Python | Java |
---|
01
02
03
04
05
06
07
08
|
def main():
x = "Bob"
print("Hello " + x)
x = 42
print(x - 1)
if __name__ == "__main__":
main()
|
|
01
02
03
04
05
06
07
08
09
10
11
|
package algs11;
import stdlib.*;
public class Hello {
public static void main (String[] args) {
Object x;
x = "Bob";
StdOut.println ("Hello " + ((String) x));
x = 42;
StdOut.println (((Integer) x) - 1);
}
}
|
|
Java will do implicit conversions in some places.
-
Try removing the casts in the code above.
The difference between the two casts occurs because every java
object can be converted to a String, using
java.util.Objects.toString
. In some contexts, this
will be done implicitly.
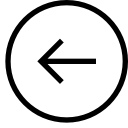
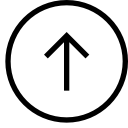
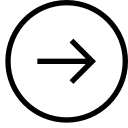