Python | Java |
---|
01
02
03
04
05
06
|
def main():
name = "Bob"
print("Hello " + name)
if __name__ == "__main__":
main()
|
|
01
02
03
04
05
06
07
08
09
|
package algs11;
import stdlib.*;
public class Hello {
public static void main (String[] args) {
String name;
name = "Bob";
StdOut.println ("Hello " + name);
}
}
|
|
In python, values are typed, but variables are not.
In java, both values and variables are typed. Variable types must
be explicitly declared.
The declaration and initialization can be combined into a single statement.
01
02
|
String name = "Bob";
StdOut.println ("Hello " + name);
|
In both languages, +
is used to represent string concatenation.
Here's another version which does not use concatenation.
Python | Java |
---|
01
02
03
04
05
06
07
|
def main():
name = "Bob"
print("Hello ", end="")
print(name)
if __name__ == "__main__":
main()
|
|
01
02
03
04
05
06
07
08
09
|
package algs11;
import stdlib.*;
public class Hello {
public static void main (String[] args) {
String name = "Bob";
StdOut.print ("Hello ");
StdOut.println (name);
}
}
|
|
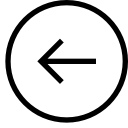
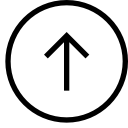
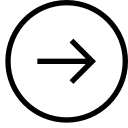