Python | Java |
---|
01
02
03
04
05
06
07
|
def main():
name = "Bob"
print("Hello ", end="")
print(name)
if __name__ == "__main__":
main()
|
|
01
02
03
04
05
06
07
08
09
|
package algs11;
import stdlib.*;
public class Hello {
public static void main (String[] args) {
String name = "Bob";
StdOut.print ("Hello ");
StdOut.println (name);
}
}
|
|
Java uses semicolons and curly-braces, where python uses newlines,
colons and indentation.
When formatting java, the conventions for indentation and newlines
mimic those of python. But in java, these are just conventions,
not requirements.
-
Try removing the newlines in the python and java code above.
If statements (aka conditionals)
Python | Java |
---|
01
02
03
04
05
06
07
08
|
def main():
i = int(input('Enter a number: '))
if i < 5:
n = 2 ** i
print (n)
if __name__ == "__main__":
main()
|
|
01
02
03
04
05
06
07
08
09
10
11
12
|
package algs11;
import stdlib.*;
public class Hello {
public static void main (String[] args) {
StdOut.print ("Enter a number: ");
int i = StdIn.readInt ();
if (i < 5) {
int n = (int) Math.pow (2, i);
StdOut.println (n);
}
}
}
|
|
Comment: Python's **
operator returns an
int
when both arguments are int
s. (If
either argument is a float
, it returns a
float
.)
Java's Math.pow
, instead, always returns a
double
. So, to recover an int
, the Java
code requires a cast.
While statements (aka loops)
Python | Java |
---|
01
02
03
04
05
06
07
08
|
def main():
i = int(input('Enter a number: '))
while i < 5:
print (i)
i = i + 1
if __name__ == "__main__":
main()
|
|
01
02
03
04
05
06
07
08
09
10
11
12
|
package algs11;
import stdlib.*;
public class Hello {
public static void main (String[] args) {
StdOut.print ("Enter a number: ");
int i = StdIn.readInt ();
while (i < 5) {
StdOut.println (i);
i = i + 1;
}
}
}
|
|
For loops
Python | Java |
---|
01
02
03
04
05
06
07
|
def main():
for i in range(int(input('Enter a number: ')), 5, -3):
n = 2 ** i
print (n)
if __name__ == "__main__":
main()
|
|
01
02
03
04
05
06
07
08
09
10
11
|
package algs11;
import stdlib.*;
public class Hello {
public static void main (String[] args) {
StdOut.print ("Enter a number: ");
for (int i = StdIn.readInt (); i > 5; i = i - 3) {
int n = (int) Math.pow (2, i);
StdOut.println (n);
}
}
}
|
|
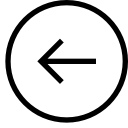
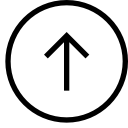
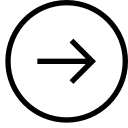